Example 7: Working with Excel
This example uses OpenPyXL to write a QPLEX pmf to an Excel spreadsheet file.
If OpenPyXL is not already installed in your working environment, do so as follows:
pip3 install openpyxl
To start, import OpenPyXL:
from openpyxl import Workbook
Then create a test pmf:
pmf = {1:0.1, 2:0.2, 3:0.3, 4:0.4}
Specify the position where you wish the pmf to be written. For example, to start in the upper left corner, do this:
initial_row = 1
initial_column = 1
Finally, specify a name for the Excel file to be created:
filename = 'demo.xlsx'
Then use standard OpenPyXL procedures to create a new spreadsheet, place the pmf in it, and save it:
wb = Workbook()
ws = wb.active
current_row = initial_row
for value, probability in pmf.items():
ws.cell(row=current_row, column=initial_column, value=value)
ws.cell(row=current_row, column=initial_column+1, value=probability)
current_row = current_row+1
wb.save(filename)
Here is the complete code:
from openpyxl import Workbook
pmf = {1:0.1, 2:0.2, 3:0.3, 4:0.4}
initial_row = 1
initial_column = 1
filename = 'demo.xlsx'
wb = Workbook()
ws = wb.active
current_row = initial_row
for value, probability in pmf.items():
ws.cell(row=current_row, column=initial_column, value=value)
ws.cell(row=current_row, column=initial_column+1, value=probability)
current_row = current_row+1
wb.save(filename)
And here is the spreadsheet it produces:
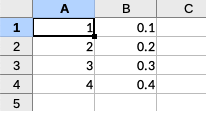